Python Elif Statement
Sometimes, the programmer may want to evaluate more than one condition, this can be done using an python elif statement.
An elif statement works on the following principle,
- execute the block of code inside if statement if the initial expression evaluates to True. After execution return to the code out of the if block.
- execute the block of code inside the first elif statement if the expression inside it evaluates True. After execution return to the code out of the if block.
- execute the block of code inside the second elif statement if the expression inside it evaluates True. After execution return to the code out of the if block….
- execute the block of code inside the nth elif statement if the expression inside it evaluates True. After execution return to the code out of the if block.
- execute the block of code inside else statement if none of the expression evaluates to True. After execution return to the code out of the if block.
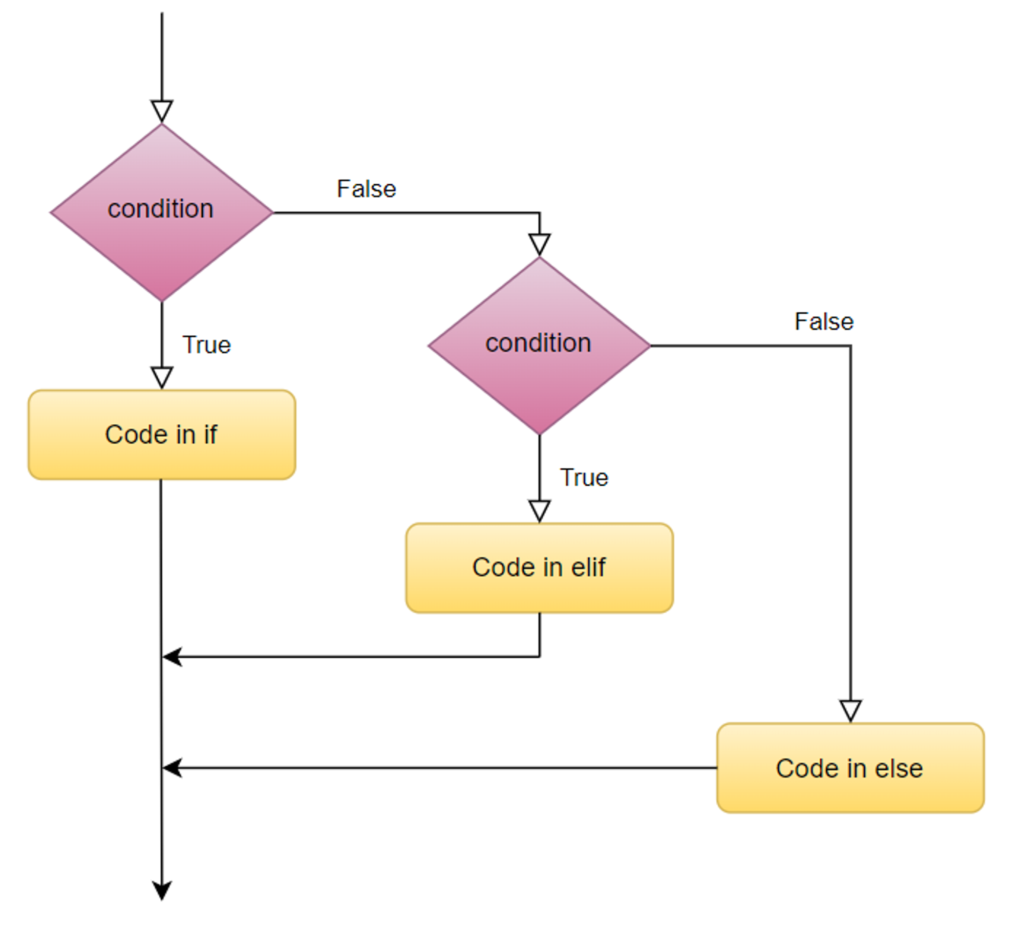
Python elif Example
num = 0
if (nuzm < 0):
print("Number is negative.")
elif (num == 0):
print("Number is Zero.")
else:
print("Number is positive.")
PythonOutput:
Number is Zero.
PythonLet’s see some real-life examples to understand how python elif
works:
- Grade Classification:
score = 75 if score >= 90: print("Grade: A") elif score >= 80: print("Grade: B") elif score >= 70: print("Grade: C") elif score >= 60: print("Grade: D") else: print("Grade: F")
In this example, based on thescore
, the program assigns a grade to a student. If the score is 75, it falls into theelif score >= 70:
condition, printing “Grade: C”. - Time of Day Greetings:
hour = 15
if hour < 12:
print("Good morning!")
elif hour < 18:
print("Good afternoon!")
else:
print("Good evening!")
PythonHere, depending on the hour
, the program greets the user appropriately. If it’s 15 (3 PM), it falls into the elif hour < 18:
condition, printing “Good afternoon!”.
- Ticket Pricing:
age = 25 is_student = False if age < 18: print("Child ticket: $8") elif age >= 65: print("Senior ticket: $10") elif is_student: print("Student ticket: $12") else: print("Standard ticket: $15")
This example determines the ticket price based on theage
and whether the person is a student. If the person is 25 years old and not a student, it falls into theelse:
condition, printing “Standard ticket: $15”.
In each of these examples, the elif
statement allows us to check additional conditions after the initial if
condition, providing a structured way to handle multiple cases in our code, similar to how we make decisions based on various conditions in real-life scenarios.
Conclusion
The elif
statement in Python is a powerful tool for building conditional logic in your programs. It allows you to handle multiple conditions in a structured and readable way. By using elif
, you can create programs that respond dynamically to different scenarios, making your code more flexible and adaptable. Remember to write clear and concise conditions, and always test your code with various inputs to ensure it behaves as expected. With practice, you’ll become proficient in using elif
statements to solve a wide range of programming problems.
Frequently Asked Questions
elif
stand for in Python? Ans: elif
stands for “else if”. It is used to check additional conditions after the initial if
condition evaluates to false.
Q2. Can I use multiple
elif
statements in a single if
block? Ans: Yes, you can have multiple elif statements in a single if block to check multiple conditions sequentially.
Q3. What happens if multiple elif conditions are true?
Ans: In Python, only the block of code associated with the first true elif condition encountered will be executed. Subsequent elif blocks are skipped.
Q4. Is elif mandatory after an if statement?
Ans: No, elif is optional. You can have just an if block without any elif or else blocks.
Q5. Can I use elif without an else block?
Ans: Yes, you can use elif without an else block. If none of the if or elif conditions are true, the program will simply continue execution after the elif block.
Q6. Can I nest elif statements within each other?
Yes, you can nest elif statements within each other to create more complex decision-making structures.
Q7. How does
elif
differ from else
? Ans: elif
is used to check additional conditions, whereas else
is used as a catch-all for conditions that are not met by the if
or elif
blocks.