Variables are critical in programming because that’s how you store data in a particular memory location. For example, a Java program, while running, stores values in containers known as “variables,” which are defined as a basic storage unit. To enhance the program’s readability, the programmer must follow particular conventions while naming these variables and assigning values to them. For example, a source code representing a fixed value is “literals in java.”
Any constant value which can be assigned to the variable is called literal/constant.
Literal in Java are a synthetic representation of boolean, character, numeric, or string data. They are a means of expressing particular values within a program. They are constant values that directly appear in a program and can be assigned now to a variable.
For Example
here is an integer variable named ‘’/count assigned as an integer value in this statement:
int count = 0;
Java“int count” is the integer variable, and a literal ‘0’ represents the value of zero.
Therefore, a constant value that is assigned to the variable can be called a literal
Types of Literals in Java
There are the majorly four types of literals in Java:
- Integer Literal
- Character Literal
- Boolean Literal
- String Literal
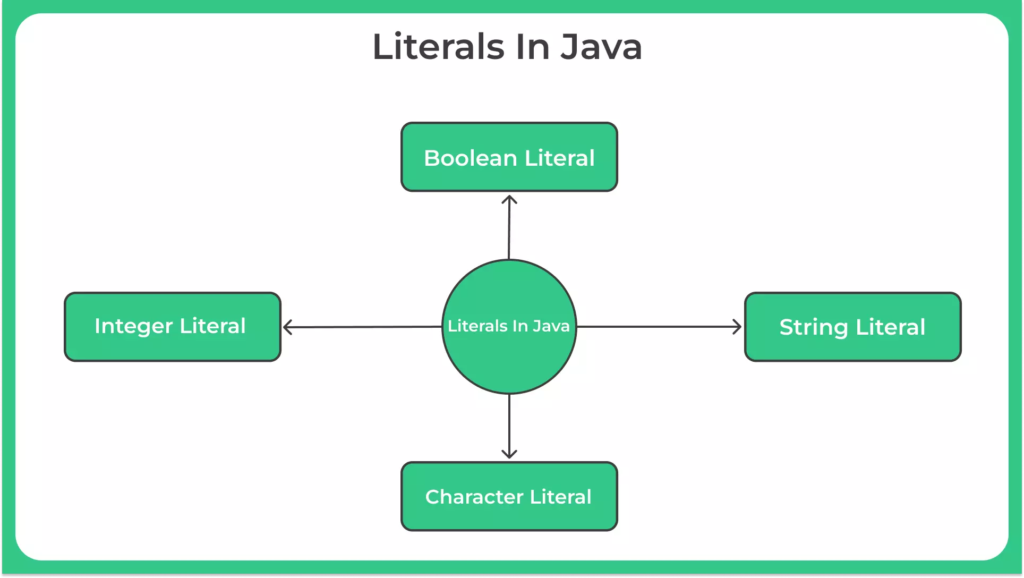
Integer Literal
Integer literals in Java are simply whole number literals without any fractional or exponential component. They can be written in decimal (base 10), octal (base 8), hexadecimal (base 16), or binary (base 2) formats.
Here are examples of integer literals in Java
1) Decimal Integer Literals:
int decimalInt = 123;
Java2) Octal Integer Literals (prefixed with 0):
int octalInt = 0123; // This represents decimal value 83 (1*8^2 + 2*8^1 + 3*8^0)
Java3 ) Hexadecimal Integer Literals (prefixed with 0x or 0X):
int hexInt = 0xABCD; // This represents decimal value 43981
Java4 ) Binary Integer Literals (Java 7 and later, prefixed with 0b or 0B):
int binaryInt = 0b101010; // This represents decimal value 42
JavaExample
public class IntegerLiteralsExample {
public static void main(String[] args) {
int decimalInt = 123;
int octalInt = 0123;
int hexInt = 0xABCD;
int binaryInt = 0b101010;
System.out.println("Decimal Integer Literal: " + decimalInt);
System.out.println("Octal Integer Literal: " + octalInt);
System.out.println("Hexadecimal Integer Literal: " + hexInt);
System.out.println("Binary Integer Literal: " + binaryInt);
}
}
JavaOutput
Decimal Integer Literal: 123
Octal Integer Literal: 83
Hexadecimal Integer Literal: 43981
Binary Integer Literal: 42
JavaCharacter Literal
Character literals in Java are represented by single characters enclosed within single quotes (‘ ‘). They can be any Unicode character, including letters, digits, special characters, and escape sequences.
Here are some examples of character literals in Java:
1.Single characters:
char letterA = 'A';
char digit5 = '5';
char specialChar = '@';
Java2.Escape sequences
char newline = 'n'; // Represents a newline character
char tab = 't'; // Represents a tab character
char backslash = '\'; // Represents a backslash character
char singleQuote = '''; // Represents a single quote character
char unicodeChar = 'u03A9'; // Represents the Greek capital letter Omega (Ω)
JavaExample
public class CharacterLiteralsExample {
public static void main(String[] args) {
char letterA = 'A';
char digit5 = '5';
char specialChar = '@';
char newline = 'n';
char tab = 't';
char backslash = '\';
char singleQuote = ''';
char unicodeChar = 'u03A9';
System.out.println("Single Characters:");
System.out.println("Letter A: " + letterA);
System.out.println("Digit 5: " + digit5);
System.out.println("Special Character: " + specialChar);
System.out.println("nEscape Sequences:");
System.out.println("Newline: " + newline);
System.out.println("Tab: " + tab);
System.out.println("Backslash: " + backslash);
System.out.println("Single Quote: " + singleQuote);
System.out.println("Unicode Character (Omega): " + unicodeChar);
}
}
JavaOutput
Single Characters:
Letter A: A
Digit 5: 5
Special Character: @
Escape Sequences:
Newline:
Tab:
Backslash:
Single Quote: '
Unicode Character (Omega): Ω
Java3 ) Boolean Literal
In Java, boolean literals represent two possible values: true
and false
. These values are keywords in the Java language and are used to denote boolean data types.
Here’s how boolean literals are used in Java:
boolean isTrue = true;
boolean isFalse = false;
JavaExample
public class BooleanLiteralsExample {
public static void main(String[] args) {
boolean isTrue = true;
boolean isFalse = false;
System.out.println("isTrue: " + isTrue);
System.out.println("isFalse: " + isFalse);
}
}
JavaOutput
isTrue: true
isFalse: false
Java4. String Literal
In Java, string literals represent sequences of characters enclosed within double quotes ("
). They are used to create string objects.
Here are some examples of string literals in Java:
String str1 = "Hello";
String str2 = "Java";
String str3 = "123";
String str4 = ""; // An empty string
String str5 = " "; // A string containing a single space
String str6 = "Hello, world!";
String str7 = "Special characters: @#$%^&*()";
String str8 = "Escaped characters: nt"\";
JavaExample
public class StringLiteralsExample {
public static void main(String[] args) {
String str1 = "Hello";
String str2 = "Java";
String str3 = "123";
String str4 = "";
String str5 = " ";
String str6 = "Hello, world!";
String str7 = "Special characters: @#$%^&*()";
String str8 = "Escaped characters: nt"\";
System.out.println("str1: " + str1);
System.out.println("str2: " + str2);
System.out.println("str3: " + str3);
System.out.println("str4: "" + str4 + "" (empty string)");
System.out.println("str5: "" + str5 + "" (single space)");
System.out.println("str6: " + str6);
System.out.println("str7: " + str7);
System.out.println("str8: " + str8);
}
}
JavaOutput
str1: Hello
str2: Java
str3: 123
str4: "" (empty string)
str5: " " (single space)
str6: Hello, world!
str7: Special characters: @#$%^&*()
str8: Escaped characters:
JavaConclusion
Java literals are fundamental elements in Java programming, representing fixed values hardcoded into the source code. They encompass various types, including integer, floating-point, character, string, boolean, and the null literal. Integer literals denote whole numbers and can be expressed in decimal, octal, hexadecimal, or binary formats. Floating-point literals represent numbers with decimal points or exponential components, while character literals denote single characters enclosed within single quotes, including escape sequences for special characters. String literals represent sequences of characters enclosed within double quotes and are used to create string objects. Boolean literals denote the boolean values true
and false
, crucial for boolean data types. Additionally, the null literal signifies the absence of a value, particularly useful for initializing object references. In Java programming, literals play a pivotal role in initializing variables, specifying values in expressions, and defining constant values throughout the codebase. Understanding and effectively utilizing Java literal are essential skills for Java developers, as they form the backbone of data representation and manipulation within Java applications.
Frequently Asked Questions
Ans: Java literal are representations of fixed values in the source code. They are hardcoded values that do not change during program execution.
Q2. What types of literal does Java support?
Ans: Java supports various types of literal, including integer literal, floating-point literal, character literal, string literal, boolean literal, and the null literal.
Q3. What are integer literal in Java?
Ans: Integer literal in Java represent whole numbers without any fractional or exponential component. They can be written in decimal, octal, hexadecimal, or binary formats.
Q4. Can you provide examples of integer literal in Java?
Ans: Certainly! Examples include 123 (decimal), 0123 (octal), 0xABCD (hexadecimal), and 0b101010 (binary).
Q5. What are floating-point literals in Java? ◦
Ans: Floating-point literal in Java represent numbers with a decimal point or exponential component. They can be specified using either decimal notation or scientific notation.
Q6. How do you represent character literals in Java?
Ans: Character literal in Java are represented by single characters enclosed within single quotes (‘ ‘). They can include normal characters or escape sequences to represent special characters.
Q7. What are string literals in Java?
Ans: String literal in Java represent sequences of characters enclosed within double quotes (” “). They are used to create string objects.
Q8. What values do boolean literal in Java represent?
Ans: Boolean literal in Java represent the two boolean values: true and false. They are used to denote boolean data types.
Q9. Is there a special literal for representing the absence of a value in Java?
Ans: Yes, in Java, the null literal is used to indicate the absence of a value. It is mainly used for initializing object references when no other value is available.
Q10. How are literals used in Java programming?
Ans: Literal are used throughout Java programming for initializing variables, specifying values in expressions, and defining constant values. They play a fundamental role in writing Java code.