The concept of a java wrapper classes has been a cornerstone of my development work. These classes, designed to convert Java’s primitive data types into reference types, have been instrumental in enabling my projects to leverage the full power of object-oriented programming. From enhancing the functionality of collections to allowing null values and providing utility methods, wrapper classes have enriched my coding experience significantly.
What is a Wrapper Classes in Java?
Java is an object-oriented language that converts a primitive data type into a class object; hence, wrapper class objects enable us to convert the original passed value. These wrapper classes support the multithreading and synchronization process. With the help of this, we can work with collections like Vector, LinkedList, and ArrayList.
Java programming language offers java.lang package that includes the Object and Class. Along with the above overview on what is wrapper class in Java, you must know what they represent. Java wrapper classes represent or wrap the primitive data types’ values as an object. When an object is defined in a wrapper class, it includes a field that can store the primitive data types’ values.
Note – That the object of one data type includes a field of the specific data type only. So, an object’s double type contains the double type of the field only. It represents that value to store the corresponding reference in a reference type’s variable.
When a wrapper class object is created, the space is allocated in the memory. This is where the primitive data type is saved. Moreover, the wrapper class supports some functionalities for transforming the object into primitive data and, eventually, primitive data into the object. These conversion processes happen automatically.
Process Flow of the Wrapper Class
In a wrapper class, we create the object with fields or properties, where we can use and store the primitive data types.
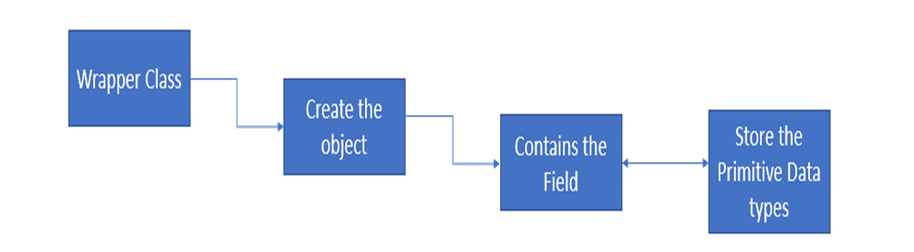
Java implements in-built classes corresponding to specific primitive types that can be applied to modify these value types in object types. We can consider and identify these inbuilt classes as wrapper classes or primitive wrapper classes.
Need of Wrapper Classes
Wrapper classes serve several important purposes in programming, particularly in languages like Java where primitive types cannot be directly used in certain situations. Here’s a list of their key roles and benefits:
- Object-oriented compatibility: In object-oriented programming, everything is treated as an object. Wrapper classes allow primitive data types to be treated as objects, enabling them to participate in object-oriented paradigms like inheritance, polymorphism, and encapsulation.
- Nullable values: Primitive types cannot represent null values, which can be essential in certain scenarios, such as when dealing with databases or APIs. Wrapper classes like
Integer
,Double
, etc., allow for nullable values by providing anull
reference. - Collections: Many collection classes in Java, such as
ArrayList
orHashMap
, require objects, not primitives. Wrapper classes allow primitive values to be stored in collections by wrapping them in objects. - Generics: Java’s generics do not support primitive types. Therefore, when you need to use generics with primitives, wrapper classes are necessary. For instance,
List<Integer>
is valid, butList<int>
is not. - Type conversions: Wrapper classes provide methods to convert between primitive types and objects. For example,
Integer.parseInt()
converts a string to anint
, andInteger.toString()
converts anInteger
object to a string. - Utility methods: Wrapper classes offer various utility methods for working with primitive values, such as comparing, sorting, and finding the minimum or maximum value.
- Interoperability: In scenarios where primitive types need to be passed to methods expecting objects, wrapper classes facilitate interoperability by allowing primitives to be wrapped and passed as arguments.
- Constants and methods: Wrapper classes often provide constants (like
Integer.MAX_VALUE
) and methods (likeInteger.valueOf()
) that are useful in various programming scenarios. - Reflection: Wrapper classes play a crucial role in reflection, allowing you to inspect and manipulate primitive types as objects during runtime.
Features of Java Wrapper Classes
- Wrapper classes transmute numeric strings into numeric values.
- We can store primitive data in an object.
- We can use the valueOf() method in all the wrapper classes without strings.
- We can use the typeValue() for all the available wrapper classes. It performs as the primitive type and returns the value of the object.
Primitive Data Types and their Corresponding Wrapper Class
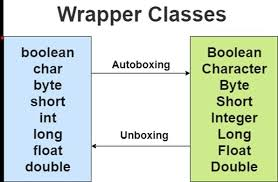
Autoboxing and Unboxing
Autoboxing and unboxing are features introduced in Java 5 to automatically convert between primitive data types and their corresponding wrapper classes. These features simplify code and make it more readable by reducing the need for manual conversion between primitives and wrappers. Here’s how they work:
- Autoboxing:
- Autoboxing is the automatic conversion of primitive types to their corresponding wrapper classes.
- For example, when assigning a primitive value to a wrapper class object, autoboxing automatically converts the primitive to its wrapper class equivalent.
- Unboxing:
- Unboxing is the automatic conversion of wrapper class objects to their corresponding primitive types.
- For example, when a wrapper class object is used in a context where a primitive type is expected, unboxing automatically extracts the primitive value from the wrapper object.
Example
public class AutoboxingUnboxingExample {
public static void main(String[] args) {
// Autoboxing: converting int to Integer
Integer intValue = 10; // Autoboxing - int to Integer
// Unboxing: converting Integer to int
int primitiveValue = intValue; // Unboxing - Integer to int
System.out.println("Autoboxing: int to Integer - intValue: " + intValue);
System.out.println("Unboxing: Integer to int - primitiveValue: " + primitiveValue);
// Autoboxing and unboxing in method parameters
calculateSquare(intValue); // Autoboxing - int to Integer
}
public static void calculateSquare(Integer num) {
// Unboxing - Integer to int
int square = num * num;
System.out.println("Square of " + num + " is: " + square);
}
}
JavaOutput
Autoboxing: int to Integer - intValue: 10
Unboxing: Integer to int - primitiveValue: 10
Square of 10 is: 100
JavaExplanation:
- The first
System.out.println
statement prints the value ofintValue
, which is10
. - The second
System.out.println
statement prints the value ofprimitiveValue
, which is also10
(after unboxing). - The
calculateSquare
method is called withintValue
as an argument. Inside the method, theInteger
value is unboxed to anint
and squared. The result,100
is printed along with the original value10
.
Java Wrapper Classes Example
public class WrapperClassesExample {
public static void main(String[] args) {
// Creating objects of wrapper classes
Integer intObj = new Integer(100); // Using constructor
Double doubleObj = Double.valueOf(3.14); // Using valueOf() method
Boolean boolObj = Boolean.TRUE; // Using predefined constant
// Autoboxing: assigning primitive values to wrapper objects
Integer anotherIntObj = 200; // Autoboxing - int to Integer
// Unboxing: extracting primitive values from wrapper objects
int intValue = intObj.intValue(); // Unboxing - Integer to int
double doubleValue = doubleObj.doubleValue(); // Unboxing - Double to double
boolean boolValue = boolObj.booleanValue(); // Unboxing - Boolean to boolean
// Printing values
System.out.println("Integer Object: " + intObj);
System.out.println("Double Object: " + doubleObj);
System.out.println("Boolean Object: " + boolObj);
System.out.println("Another Integer Object: " + anotherIntObj);
System.out.println("Unboxed Integer Value: " + intValue);
System.out.println("Unboxed Double Value: " + doubleValue);
System.out.println("Unboxed Boolean Value: " + boolValue);
// Using wrapper classes in collections
java.util.ArrayList<Integer> arrayList = new java.util.ArrayList<>();
arrayList.add(10); // Autoboxing - int to Integer
arrayList.add(20); // Autoboxing - int to Integer
System.out.println("ArrayList: " + arrayList);
}
}
JavaOutput
Integer Object: 100
Double Object: 3.14
Boolean Object: true
Another Integer Object: 200
Unboxed Integer Value: 100
Unboxed Double Value: 3.14
Unboxed Boolean Value: true
ArrayList: [10, 20]
JavaExplanation:
Integer
,Double
, andBoolean
wrapper objects are created with values100
,3.14
, andtrue
respectively.- Autoboxing is demonstrated when assigning
200
toanotherIntObj
. - Unboxing occurs when extracting primitive values from wrapper objects (
intValue
,doubleValue
,boolValue
). - Values of all wrapper objects and unboxed primitive values are printed.
- Wrapper objects are used in an
ArrayList
, and the contents of theArrayList
are printed.
Custom Wrapper Classes in Java
Custom wrapper classes in Java are classes that wrap around primitive types or other objects, providing additional functionality or behavior as needed. Below is an example of a custom wrapper class that wraps around an int
value and provides methods for incrementing and decrementing the value.
Example
public class CustomIntWrapper {
private int value;
// Constructor
public CustomIntWrapper(int value) {
this.value = value;
}
// Getter method
public int getValue() {
return value;
}
// Setter method
public void setValue(int value) {
this.value = value;
}
// Method to increment value by one
public void increment() {
value++;
}
// Method to decrement value by one
public void decrement() {
value--;
}
// Method to add a specific value
public void add(int num) {
value += num;
}
// Method to subtract a specific value
public void subtract(int num) {
value -= num;
}
// toString method for string representation
@Override
public String toString() {
return "CustomIntWrapper{" +
"value=" + value +
'}';
}
public static void main(String[] args) {
// Create an instance of CustomIntWrapper
CustomIntWrapper wrapper = new CustomIntWrapper(10);
// Print initial value
System.out.println("Initial Value: " + wrapper);
// Perform operations
wrapper.increment();
System.out.println("After increment: " + wrapper);
wrapper.add(5);
System.out.println("After adding 5: " + wrapper);
wrapper.subtract(3);
System.out.println("After subtracting 3: " + wrapper);
}
}
JavaOutput
Initial Value: CustomIntWrapper{value=10}
After increment: CustomIntWrapper{value=11}
After adding 5: CustomIntWrapper{value=16}
After subtracting 3: CustomIntWrapper{value=13}
JavaExplanation:
- Initially, the
CustomIntWrapper
object is created with a value of10
. - After each operation (
increment
,add
,subtract
), the value of theCustomIntWrapper
object is updated accordingly, and the object’s string representation is printed.
Advantages and Disadvantages of Wrapper Class in Java
Advantages
- Object-oriented compatibility: Wrapper classes allow primitive data types to be treated as objects, facilitating their use in object-oriented paradigms like inheritance and polymorphism.
- Nullable values: Wrapper classes can represent
null
values, which is not possible with primitive types alone. This is useful in scenarios where nullability needs to be explicitly handled, such as database operations. - Generics support: Java generics do not support primitive types, so wrapper classes are necessary when using generics with primitives. For example,
List<Integer>
is valid, butList<int>
is not. - Collections: Many collection classes in Java (e.g.,
ArrayList
,HashMap
) require objects, not primitives. Wrapper classes allow primitive values to be stored in collections by wrapping them in objects. - Utility methods: Wrapper classes offer utility methods for working with primitive values, such as comparing, sorting, and converting between types.
- Interoperability: Wrapper classes enable interoperability by allowing primitives to be wrapped and passed as arguments to methods expecting objects.
Disadvantages
- Performance overhead: Wrapper classes can introduce performance overhead due to the additional memory consumption and method invocation overhead associated with working with objects.
- Memory consumption: Wrapper objects typically consume more memory than their primitive counterparts, as they carry additional metadata and overhead.
- Autoboxing/unboxing overhead: Autoboxing (converting primitive types to wrapper objects) and unboxing (extracting primitive values from wrapper objects) can lead to overhead in terms of CPU cycles and memory usage, especially in performance-sensitive applications.
- Potential for NullPointerException: When using wrapper classes, there is a risk of encountering
NullPointerException
if proper null checks are not performed, especially when working with autoboxing or methods that return wrapper objects. - Complexity: Introducing wrapper classes can sometimes add complexity to the code, especially in scenarios where autoboxing/unboxing is mixed with manual conversion between primitive types and wrapper objects.
Conclusion
Wrapper classes in Java serve as essential tools for bridging the gap between primitive types and objects. They offer several advantages such as enabling object-oriented compatibility, supporting nullable values, facilitating generics usage, providing utility methods, and enhancing interoperability. However, their usage comes with certain disadvantages including potential performance overhead, increased memory consumption, autoboxing/unboxing overhead, risk of NullPointerException, and added complexity to the code.
When considering the use of wrapper classes in Java, it’s crucial to weigh these pros and cons against the specific requirements and constraints of the application. While wrapper classes provide valuable functionality, their usage should be balanced with considerations for performance, memory usage, and code complexity. By carefully evaluating these factors, developers can effectively leverage wrapper classes to enhance their Java applications while ensuring optimal performance and maintainability.
Frequently Asked Questions
Wrapper classes are classes that encapsulate primitive data types and provide methods to manipulate them as objects. They allow primitive types to be used in scenarios where objects are required, such as collections, generics, and method overloading.
Wrapper classes enable object-oriented compatibility, support nullable values, facilitate generics usage, provide utility methods, and enhance interoperability between primitive types and objects.
Wrapper classes are useful when working with collections, generics, APIs that expect objects, and scenarios where nullability needs to be explicitly handled. They also come in handy for performing operations that require object-oriented features like inheritance and polymorphism.
Wrapper classes may introduce performance overhead due to increased memory consumption, autoboxing/unboxing overhead, and additional method invocation overhead. However, the impact on performance can vary depending on the specific use case and should be considered when designing performance-sensitive applications.