Java programming language has a rich set of data types. The Java programming language is statically-typed, which means that all variables must first be declared before they can be used. The data type is a category of data stored in variables. The use of data types is crucial for ensuring data integrity and efficient memory usage in Java programs.
Data Types Are Classified Into Two Types
• Primitive Data Types • Non-primitive Data Types
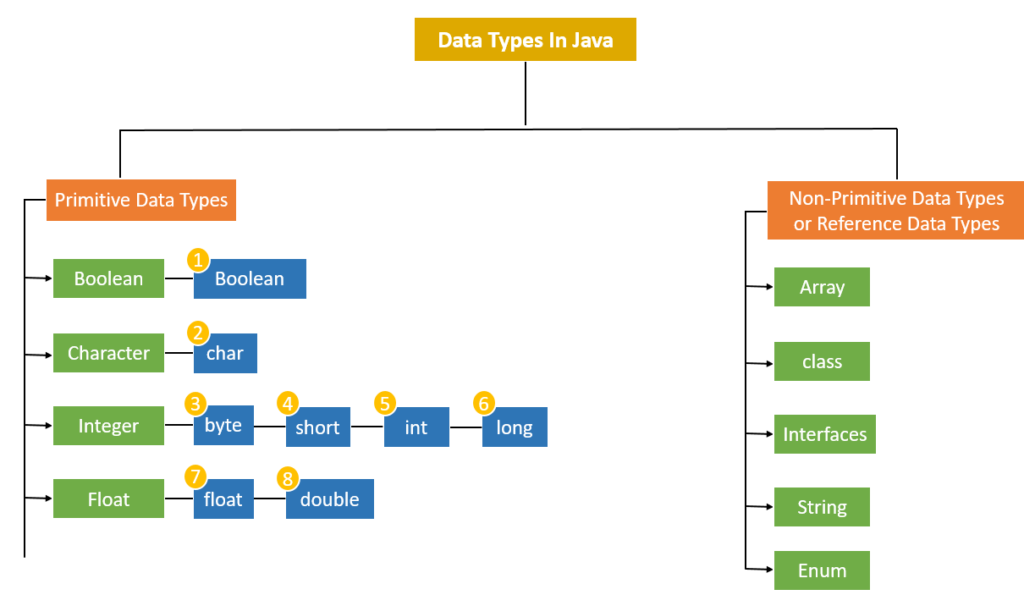
Primitive Data Types Table – Default Value, Size, and Range
Data Type | Default Value | Default size | Range |
byte | 0 | 1 byte or 8 bits | -128 to 127 |
short | 0 | 2 bytes or 16 bits | -32,768 to 32,767 |
int | 0 | 4 bytes or 32 bits | 2,147,483,648 to 2,147,483,647 |
long | 0 | 8 bytes or 64 bits | 9,223,372,036,854,775,808 to 9,223,372,036,854,775,807 |
float | 0.0f | 4 bytes or 32 bits | 1.4e-045 to 3.4e+038 |
double | 0.0d | 8 bytes or 64 bits | 4.9e-324 to 1.8e+308 |
char | ‘u0000’ | 2 bytes or 16 bits | 0 to 65536 |
boolean | FALSE | 1 byte or 2 bytes | 0 or 1 |
These Eight Primitive Data Types Can Be Put Into Four Groups:
- Integers This group includes byte, short, int, and long, which are for whole-valued signed numbers.
- Floating-point numbers – This group includes float and double, which represent numbers with fractional precision.
- Characters This group includes char, which represents symbols in a character set, like letters and numbers.
- Boolean This group includes boolean, which is a special type for representing true/false values.
Let’s discuss each primitive datatype with examples.
1. Integers
Java defines four integer types: byte, short, int, and long. All of these are signed, positive and negative values. Java does not support unsigned, positive-only integers.
Let’s look at each type of integer.
i. Byte
The byte data type is an example of primitive data type. It isan 8-bit signed two’s complement integer. Its value-range lies between -128 to 127 (inclusive). Its minimum value is -128 and maximum value is 127. Its default value is 0.
The byte data type is used to save memory in large arrays where the memory savings is most required. It saves space because a byte is 4 times smaller than an integer. It can also be used in place of “int” data type.
Example:
byte a = 10;
byte b = -20;
Javaii. Short
The short data type is a 16-bit signed two’s complement integer. Its value-range lies between -32,768 to 32,767 (inclusive). Its minimum value is -32,768 and maximum value is 32,767. Its default value is 0.
The short data type can also be used to save memory just like byte data type. A short data type is 2 times smaller than an integer.
Example:
- short s = 10000, short r = -5000
iii. int
The int data type is a 32-bit signed two’s complement integer. Its value-range lies between – 2,147,483,648 (-2^31) to 2,147,483,647 (2^31 -1) (inclusive). Its minimum value is – 2,147,483,648and maximum value is 2,147,483,647. Its default value is 0.
The int data type is generally used as a default data type for integral values unless if there is no problem about memory.
Example:
- int a = 100000, int b = -200000
iv. Long
The long data type is a 64-bit two’s complement integer. Its value-range lies between -9,223,372,036,854,775,808(-2^63) to 9,223,372,036,854,775,807(2^63 -1)(inclusive). Its minimum value is – 9,223,372,036,854,775,808and maximum value is 9,223,372,036,854,775,807. Its default value is 0. The long data type is used when you need a range of values more than those provided by int.
Example:
- long a = 100000L, long b = -200000L
2. Floating-Point Types
Floating-point numbers, also known as real numbers, are used when evaluating expressions that require fractional precision. For example, calculations such as square root, or transcendentals such as sine and cosine, result in a value whose precision requires a floating-point type.
i. Float
The float data type is a single-precision 32-bit IEEE 754 floating point.Its value range is unlimited. It is recommended to use a float (instead of double) if you need to save memory in large arrays of floating point numbers. The float data type should never be used for precise values, such as currency. Its default value is 0.0F.
Example:
- float f1 = 234.5f
ii. Double
The double data type is a double-precision 64-bit IEEE 754 floating point. Its value range is unlimited. The double data type is generally used for decimal values just like float. The double data type also should never be used for precise values, such as currency. Its default value is 0.0d.
Example:
1. double d1 = 12.3
3. Characters
i. Char
The char data type is a single 16-bit Unicode character. Its value-range lies between ‘u0000’ (or 0) to ‘uffff’ (or 65,535 inclusive).The char data type is used to store characters.
Example:
char letterA = 'A'
Java4. Booleans
i. Boolean
The Boolean data type is used to store only two possible values: true and false. This data type is used for simple flags that track true/false conditions.
The Boolean data type specifies one bit of information, but its “size” can’t be defined precisely.
Example:
Boolean one = false;
JavaWhy char uses 2 byte in JAVA and What is u0000 ?
In Java, a char
data type uses 2 bytes because it represents a Unicode character, and Unicode characters are typically encoded in 16 bits, which corresponds to 2 bytes. This allows Java to support a wide range of characters from different languages and character sets.
The u0000
is a Unicode escape sequence in Java. It represents the null character, which has a Unicode value of 0. This escape sequence allows you to represent Unicode characters in your Java code using their hexadecimal Unicode values. For example, u0041
represents the uppercase letter ‘A’, and u03B1
represents the Greek letter alpha.
Here’s an example code that demonstrates all the primitive data types in Java:
public class PrimitiveTypesExample {
public static void main(String[] args) {
byte myByte = 100;
short myShort = 1000;
int myInt = 1000000;
long myLong = 1000000000L;
float myFloat = 3.14f;
double myDouble = 3.14159265359;
boolean myBoolean = true;
char myChar = 'A';
System.out.println("byte: " + myByte);
System.out.println("short: " + myShort);
System.out.println("int: " + myInt);
System.out.println("long: " + myLong);
System.out.println("float: " + myFloat);
System.out.println("double: " + myDouble);
System.out.println("boolean: " + myBoolean);
System.out.println("char: " + myChar);
}
}
JavaNon-Primitive Data Types
Non-primitive data types or reference data types refer to instances or objects. They cannot store the value of a variable directly in memory. They store a memory address of the variable. Unlike primitive data types we define by Java, non-primitive data types are user-defined. Programmers create them and can be assigned with null. All non-primitive data types are of equal size.
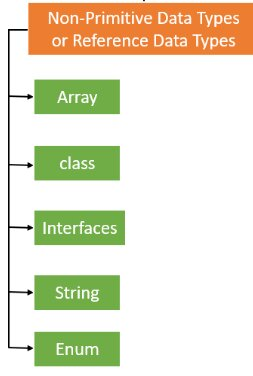
1. Arrays:
A collection of similar types of data. For example, int[] arr = new int[5];
.
2. Classes:
User-defined data types. For example, String
, ArrayList
, etc.
3. Interfaces:
Like classes but only contain method signatures. For example, Comparable
, Serializable
, etc.
4. String :
In Java, a string is a sequence of characters. It’s a data type used to represent text rather than numeric data. Strings in Java are immutable, meaning once a string object is created, its contents cannot be changed.
Example :
// Declare String without using new operator
String s = "Welcome to Geekster !";
// Declare String using new operator
String s1 = new String("Welcome to Geekster !");
Java5. Enum:
An enum is a special data type used to define a collection of constants. It allows you to create a set of named constants that represent a finite set of possibilities, typically related to some specific type or category. Enums are declared using the enum
keyword.
Example :
enum Day {
SUNDAY,
MONDAY,
TUESDAY,
WEDNESDAY,
THURSDAY,
FRIDAY,
SATURDAY
}
Java- Primitive data types are passed by value, meaning the actual value is passed to methods. Reference data types, on the other hand, are passed by reference, meaning a reference (memory address) to the object is passed to methods.
Conclusion
Java data types are essential components of Java programming, providing a structured approach to handling data within applications. Java offers versatility and flexibility in data manipulation.Understanding Java data types is crucial for efficient memory management, type safety, portability, performance optimization, and ensuring compatibility across different Java implementations.
Overall, a solid grasp of Java data types empowers developers to write reliable, maintainable, and platform-independent code that meets the demands of modern software development.
Frequently Asked Questions
Ans: Java datatypes are used to define the type of data a variable can hold. They specify the size and type of values that can be stored in variables.
Q2. What are the different types of Java datatypes?
Ans: Java datatypes are categorized into two main groups: primitive datatypes and reference datatypes. Primitive datatypes include byte, short, int, long, float, double, char, and boolean. Reference datatypes include classes, interfaces, arrays, and enumerations.
Q3. What are primitive datatypes?
Ans: Primitive datatypes are basic data types provided by Java, and they are not objects. They include byte, short, int, long, float, double, char, and boolean.
Q4. What are reference datatypes?
Ans: Reference datatypes are data types that refer to objects in memory. They include classes, interfaces, arrays, and enumerations.
Q5. What is the difference between primitive and reference datatypes?
Ans: Primitive datatypes store actual values, whereas reference datatypes store references (memory addresses) to objects.
Q6. How are Java datatypes used in variable declaration?
Ans: Java datatypes are used when declaring variables to specify the type of data the variable can hold. For example: int myNumber = 10; Here, int is the datatype specifying that myNumber can hold integer values.
Q7. What is autoboxing and unboxing in Java?
Ans: Autoboxing is the automatic conversion of primitive datatypes to their corresponding wrapper class objects (e.g., int to Integer). Unboxing is the reverse process, where wrapper class objects are automatically converted to their corresponding primitive datatypes.
Q8. How are Java datatypes used in method parameters and return types?
Ans: Java datatypes are used to specify the types of parameters that a method can accept and the type of value that the method can return. For example: public void printNumber(int num) specifies that the method printNumber accepts an integer parameter.
Q9. Can Java datatypes be converted from one type to another?
Ans: Yes, Java provides methods for converting between different datatypes. For example, you can use type casting to convert from one primitive datatype to another, or you can use methods like parseInt()
to convert a string to an integer.
Q10. Are there any limitations or considerations when using Java datatypes?
Ans: While Java provides a wide range of datatypes to suit various needs, it’s important to consider factors such as memory usage, precision, and performance when choosing datatypes for variables and operations. Additionally, care should be taken when converting between datatypes to avoid loss of data or unexpected behavior.