Introduction
if-else
statement in C++ are fundamental control structures used to make decisions and execute different blocks of code based on certain conditions. These statements allow the program to choose a path of execution depending on whether a condition evaluates to true
or false
.
Basic Structure of If Statement
The simplest form of an if
statement checks a condition and executes a block of code if the condition is true
:
if (condition) {
// Code to execute if condition is true
}
C++Adding an Else Clause
An else
clause can be added to execute a block of code when the if
condition is false
:
if(boolean_expression) {
// statement(s) will execute if the boolean expression is true
} else {
// statement(s) will execute if the boolean expression is false
}
C++Using Else If-else for Multiple Conditions
To handle multiple conditions, you can use the else if
statement. This allows you to test additional conditions if the initial if
condition is false
:
if (condition1) {
// Code to execute if condition1 is true
} else if (condition2) {
// Code to execute if condition1 is false and condition2 is true
} else {
// Code to execute if both condition1 and condition2 are false
}
C++How If-else Statements Work
- Evaluation of Conditions: The
if
statement evaluates the specified condition. If the condition istrue
, the code block within theif
statement executes. If the condition isfalse
, the program skips to theelse if
orelse
part, if available. - Sequential Checks: In an
if-else if-else
chain, each condition is checked in sequence. The first condition that evaluates totrue
has its associated block executed, and the remaining conditions are skipped. - Default Action: The
else
clause serves as a default action that executes when none of the preceding conditions aretrue
.
Flow chart of If else Statement
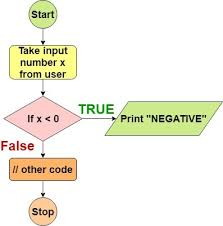
Real World Example of If else statement
Scenario: Calculating Shipping Cost Based on Package Weight
In a shipping service, the cost might be determined as follows:
- Free shipping for packages weighing 5 kg or less.
- $10 shipping for packages weighing more than 5 kg but less than or equal to 20 kg.
- $25 shipping for packages weighing more than 20 kg.
C++ Code Example
#include <iostream>
using namespace std;
int main() {
double weight;
cout << "Enter the package weight in kg: ";
cin >> weight;
double shippingCost;
if (weight <= 5) {
shippingCost = 0.0;
} else if (weight <= 20) {
shippingCost = 10.0;
} else {
shippingCost = 25.0;
}
cout << "The shipping cost is: $" << shippingCost << endl;
return 0;
}
C++Explanation
- Input Package Weight: The program prompts the user to enter the weight of the package.
- Determine Shipping Cost: Using
if-else
statements, the program determines the shipping cost based on the weight:- Free shipping if the weight is 5 kg or less.
- $10 if the weight is more than 5 kg but less than or equal to 20 kg.
- $25 if the weight is more than 20 kg.
- Output the Shipping Cost: The program outputs the calculated shipping cost.
Example 1
Enter the package weight in kg: 3
The shipping cost is: $0
C++Example 2
Enter the package weight in kg: 15
The shipping cost is: $10
C++Example 3
Enter the package weight in kg: 25
The shipping cost is: $25
C++This example shows how if-else
statements can be used to make decisions based on conditions and execute different actions accordingly.
Examples of If else statement
- Checking if a number is positive, negative, or zero
#include <iostream>
using namespace std;
int main() {
int num;
cout << "Enter a number: ";
cin >> num;
if (num > 0) {
cout << "The number is positive." << endl;
} else if (num < 0) {
cout << "The number is negative." << endl;
} else {
cout << "The number is zero." << endl;
}
return 0;
}
C++2.Determining if a number is even or odd
#include <iostream>
using namespace std;
int main() {
int num;
cout << "Enter a number: ";
cin >> num;
if (num % 2 == 0) {
cout << "The number is even." << endl;
} else {
cout << "The number is odd." << endl;
}
return 0;
}
C++3.Checking eligibility for voting based on age
#include <iostream>
using namespace std;
int main() {
int age;
cout << "Enter your age: ";
cin >> age;
if (age >= 18) {
cout << "You are eligible to vote." << endl;
} else {
cout << "You are not eligible to vote yet." << endl;
}
return 0;
}
C++4.Determining the largest of three numbers
#include <iostream>
using namespace std;
int main() {
int num1, num2, num3;
cout << "Enter three numbers: ";
cin >> num1 >> num2 >> num3;
if (num1 >= num2 && num1 >= num3) {
cout << "The largest number is: " << num1 << endl;
} else if (num2 >= num1 && num2 >= num3) {
cout << "The largest number is: " << num2 << endl;
} else {
cout << "The largest number is: " << num3 << endl;
}
return 0;
}
SQLConclusion
In conclusion, the if-else
statement in C++ is a fundamental control structure that allows for conditional execution of code based on certain conditions. It provides a way to make decisions within a program, allowing for branching behavior based on the evaluation of expressions.
Key points about if-else
statements include:
- Syntax: The syntax of the
if-else
statement consists of theif
keyword followed by a condition in parentheses, followed by a block of code to be executed if the condition is true. Optionally, anelse
block can follow, containing code to be executed if the condition is false. - Condition Evaluation: The condition within the
if
statement is evaluated, and if it results in a true value, the code within the corresponding block is executed. If the condition is false, and there is anelse
block, the code within theelse
block is executed. - Nested
if-else
:if-else
statements can be nested within each other to handle more complex decision-making scenarios. - Chaining
if-else
: Multipleif-else
statements can be chained together usingelse if
to handle multiple possible conditions. - Default Behavior: It’s important to consider providing default behavior or handling unexpected cases with
else
orelse if
clauses to ensure the program behaves as expected in all scenarios. - Code Readability: Proper indentation and formatting enhance the readability of
if-else
statements, making the code easier to understand and maintain.
Overall, if-else
statements are essential for implementing conditional logic in C++ programs, enabling developers to create dynamic and responsive applications that can adapt their behavior based on different input conditions.
Frequently Asked Question
A: An if-else statement is a control structure in C++ that allows for conditional execution of code. It evaluates a condition and executes a block of code if the condition is true, otherwise, it executes an alternative block of code.
A: You can write a simple if-else statement in C++ by using the if
keyword followed by a condition in parentheses, and then the code block to execute if the condition is true. Optionally, you can include an else
block with an alternative code block.
A: Yes, you can nest if-else statements within each other in C++. This allows for handling more complex decision-making scenarios where multiple conditions need to be evaluated.
A: In a series of if-else statements, only the code block corresponding to the first true condition encountered will be executed. Once a condition is true, the execution skips to the end of the entire if-else structure.