Introduction
A React component takes an optional input and returns a React element which is rendered on the screen.
A React component can be either “stateful” or “stateless.”
“Stateful” components are of the class type, while “stateless” components are of the function type.
There are two types of components:
- Class Based Components
- Function Based Components
Class Based Components
Before making class based component we need to inherit functions from React.Component
and this can be done with extends
, like this:
class Cat extends React.Component {
render() {
return <h1>Meow</h1>;
}
}
JavaScriptit also requires a render
method which returns HTML.
Function Based Components
In function it’s simpler, we just need to return the HTML, like this:
function Cat() {
return <h1>Meow</h1>;
}
JavaScriptNote: Component’s name must start with uppercase letter.
Introducing Components in JSX
- Components in JSX are like building blocks for creating the user interface.
- In JSX, components are reusable, self-contained pieces of UI.
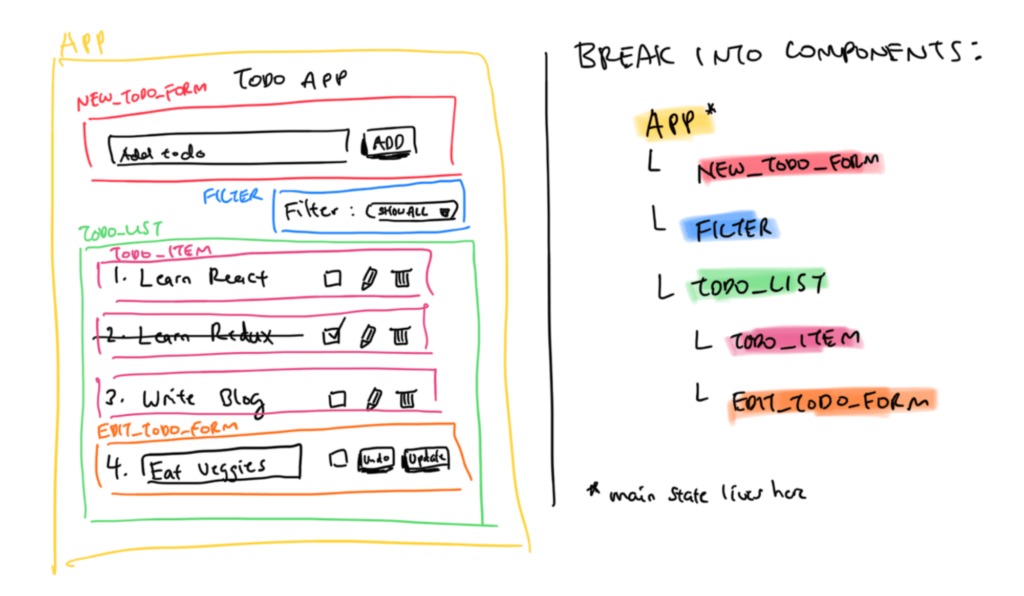
Example: Defining and using a simple component in JSX.
function Greeting() {
return <h1>Hello, Facebook!</h1>;
}
function App() {
return (
<div>
<Greeting />
</div>
);
}
JavaScriptHow Components Help
- Components make your UI code more modular, maintainable, and easier to understand.
- They enable you to reuse and compose UI elements efficiently.
- Example: Reusing a
Button
component across the app.
function Button(props) {
return <button>{props.label}</button>;
}
function App() {
return (
<div>
<Button label="Like" />
<Button label="Comment" />
</div>
);
}
JavaScriptWhat is Component and its Purpose(Functional Component):
- A component is a JavaScript function that returns JSX.
- Functional components are a type of component that is defined as a JavaScript function.
- They are used to render UI elements.
- Example: A functional component for a user profile.
function UserProfile(props) {
return (
<div>
<img src={props.avatar} alt="User Avatar" />
<h2>{props.name}</h2>
</div>
);
}
JavaScriptDifference between Component and JS Function
- Components are a specialized type of JS function for building UI.
- Regular JS functions can perform any task, while components are tailored for rendering UI.
- Components return JSX, which defines the structure of the UI.
- Example: A regular JS function vs. a component.
// Regular JS function
function add(a, b) {
return a + b;
}
// Component (Functional)
function Greeting() {
return <h1>Hello, Facebook!</h1>;
}
JavaScriptRendering the Component
- Rendering a component means displaying it on the screen.
- In React, you typically use
ReactDOM.render
to render your app’s root component. - Example: Rendering the
App
component.
ReactDOM.render(<App />, document.getElementById('root'));
JavaScriptCreating Multiple Components for Different Scenarios
- In a complex application like Facebook.com, you’d create various components for different parts of the UI.
- Each component handles a specific part of the user interface, like the news feed, chat, or user profiles.
- Example: Defining separate components for the news feed and user profile.
function NewsFeed() {
// Render news feed content
}
function UserProfile() {
// Render user profile information
}
JavaScriptComponents are at the heart of React’s component-based architecture, and they allow you to build complex UIs while keeping your code organized and maintainable. They play a crucial role in structuring the user interface of websites like Facebook.com.
Conclusion
In conclusion, components are fundamental to building modular, maintainable, and scalable user interfaces in React. By breaking down UI elements into reusable components, developers can create a clear and organized codebase that promotes code reuse and separation of concerns. Whether they are simple presentational components or complex container components managing state and behavior, each component plays a specific role in defining the UI and functionality of the application.
Frequently Asked Question
Components are the building blocks of React applications. They are reusable, self-contained units of UI that encapsulate a specific piece of functionality. Components can be simple, representing a button or an input field, or complex, representing entire sections of a web page.
React components can be classified into two main types: functional components and class components. JavaScript functions define functional components, which are stateless, while ES6 classes extending React.Component define stateful class components.
Components can communicate with each other by passing data through props (properties) or by using a state management library like Redux or context API. Parent components can pass data down to child components via props, while child components can communicate with parent components using callback functions passed as props.