Introduction
In the fascinating world of computer programming, every piece of information needs a special place to reside. Just like you have your own room to store your belongings, variables in programming have their own designated spaces called data types. C++ is a versatile programming language that offers a wide range of data types, each designed to hold specific kinds of information. Let’s embark on an exciting journey to explore these diverse data types and unlock their secrets!
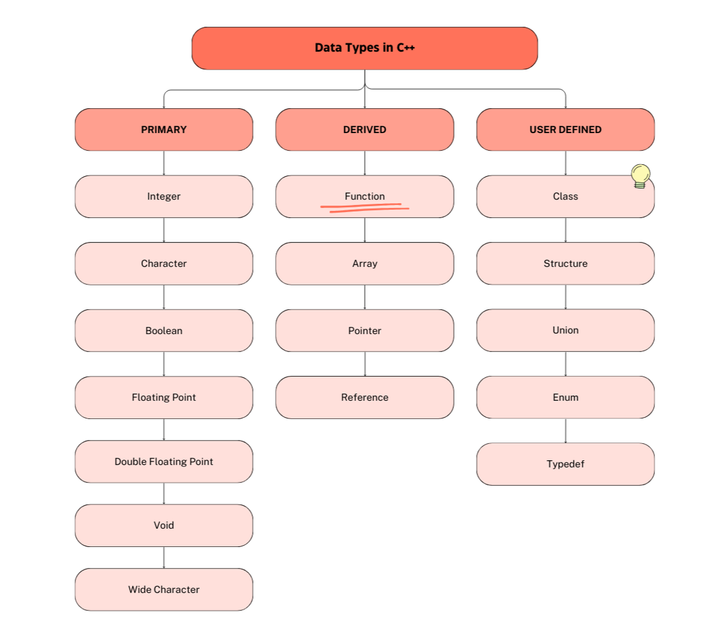
What are Data Types?
Data types are like different-sized containers or lockers that can hold various kinds of information in a program. Each data type in C++ is designed to store specific data like whole numbers, decimals, characters, or true/false values. Just like toys don’t belong in a shoe locker, data needs the right type in programming.
Primitive Data Types in C++:
C++ provides several built-in or primitive data types, each with its own unique characteristics. Here are some of the most commonly used ones:
- Integer Types (int, short int, long int): These data types are perfect for storing whole numbers, both positive and negative. Think of them as lockers that can hold your collection of action figures or trading cards.
- Floating-Point Types (float, double): If you need to store numbers with decimal places, like your allowance or the price of a toy, floating-point types are the way to go. They’re like lockers designed to hold items with various shapes and sizes.
- Character Types (char, wchar_t): Designed to store individual characters, such as letters, numbers, or special symbols. They’re like tiny lockers that can hold a single action figure or a small toy.
- Boolean Type (bool): This data type is a true or false champion! It’s like a locker that can only hold two items: a red ball (true) or a blue ball (false). It’s perfect for storing yes/no or on/off kinds of information.
Derived Data Types:
Derived data types are created from primitive or built-in data types. There are four main types:
- Function
- Array
- Pointer
- Reference
Abstract or User-Defined Data Types:
These data types are defined by the user. Examples include defining a class or a structure in C++. C++ supports the following user-defined data types:
- Class
- Structure
- Union
- Enumeration
- Typedef-defined datatype
Conclusion
Mastering data types in C++ is like having a well-organized locker room, where you can store and retrieve information efficiently. By understanding the different data types and their capabilities, you’ll be able to write more powerful and versatile programs. Whether you’re storing numbers, characters, or even true/false values, C++ has a data type that fits your needs perfectly. So, embrace the power of data types, and let your programming skills shine!
Frequently Asked Questions
No, you cannot change the data type of a variable once it has been declared and initialized in C++. If you need to store a different type of data, you’ll have to create a new variable with the desired data type.
Signed integers can store both positive and negative values, while unsigned integers can only store non-negative values. However, unsigned integers have a larger positive range than their signed counterparts because they don’t need to reserve memory for negative values.
Yes, each data type in C++ has a specific range of values it can represent, depending on the amount of memory allocated for that type. For example, an int typically has a range of around -2 billion to +2 billion, while a long long int can represent much larger values.